Schema-First Full‑Stack Toolkit
with Clean & Scalable Authorization
A TypeScript toolkit that enhances Prisma ORM with flexible Authorization and auto-generated, type-safe APIs/hooks, simplifying full-stack development
Built Above Prisma, More Than ORM
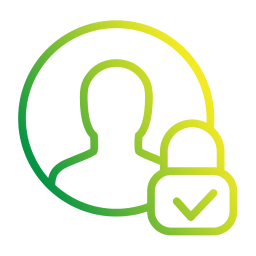
Easy Access Control
Access control policies right inside your data model. No more brittle imperative authorization code. No more complex SQL Row-Level-Security rules.
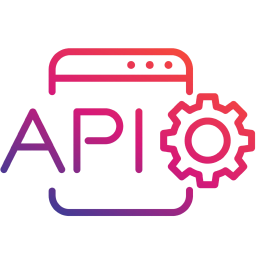
Generated API & Hooks
CRUD APIs and frontend hooks are automatically generated. With access control support, the APIs are safe to be called directly from the frontend.
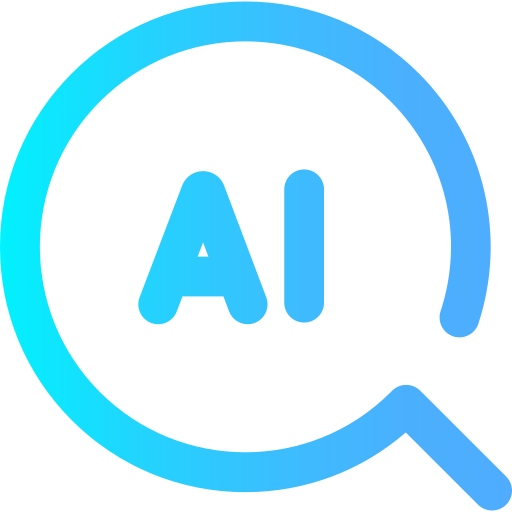
AI Friendly
Schema-first reduces code complexity, helping AI understand better with fewer hallucinations. Schema serves as a single source of truth for AI integration.
Used and Loved by
Empower Every Layer of Your Stack
ORM With Access Control
ZenStack extends Prisma ORM with a powerful access control layer. By defining policies right inside the data model, your schema becomes the single source of truth. By using a policy-enabled database client, you can enjoy the same Prisma API you already love, with ZenStack automagically enforcing access control rules. Its core is framework-agnostic and runs wherever Prisma runs.
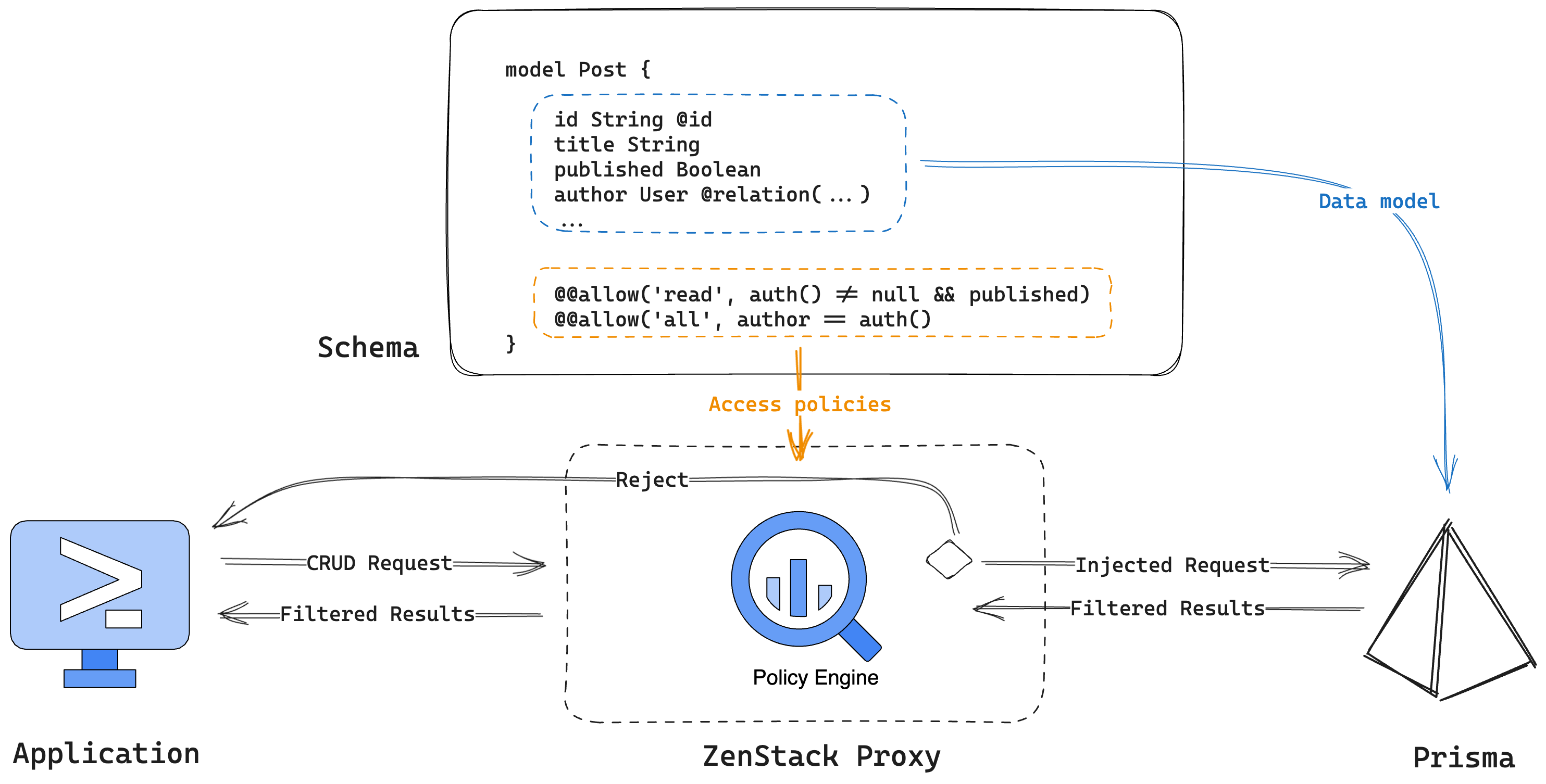
Automatic CRUD API
Wrapping APIs around a database is tedious and error-prone. ZenStack can introspect the schema and install CRUD APIs to the framework of your choice with just a few lines of code. Thanks to the built-in access control support, the APIs are fully secure and can be directly exposed to the public. What about documentation? Turn on a plugin, and an OpenAPI specification will be generated in seconds.
- Next.js
- Nuxt
- SvelteKit
- Express
- Fastify
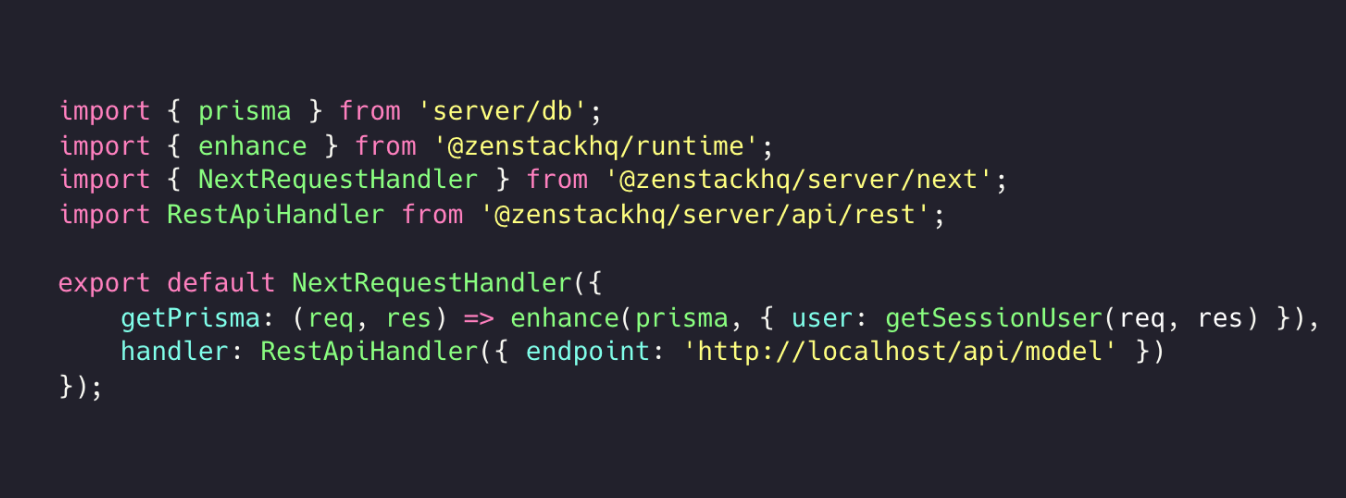
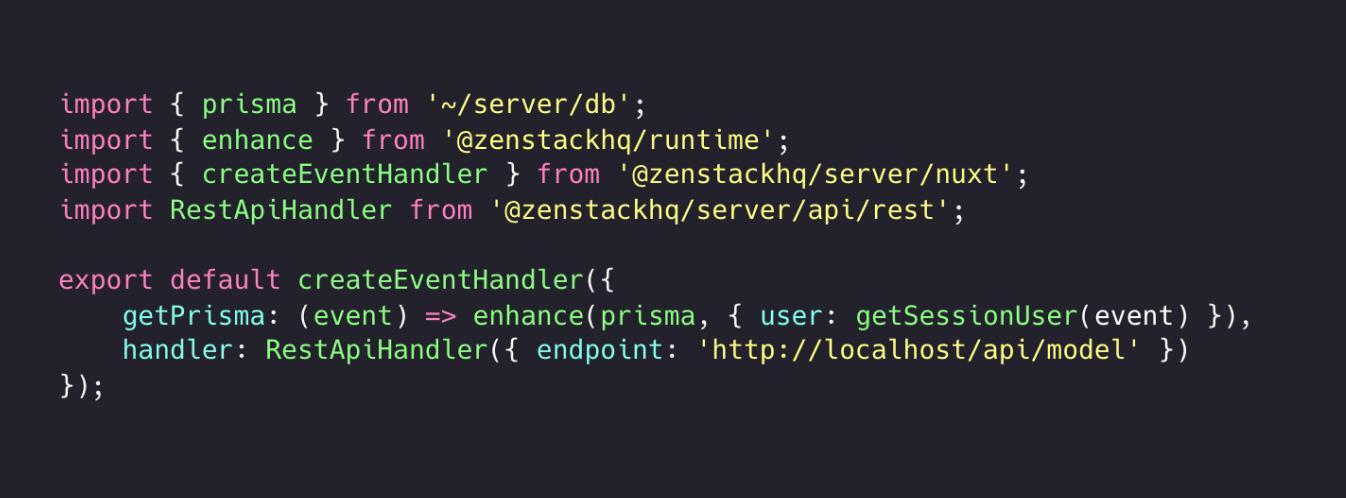
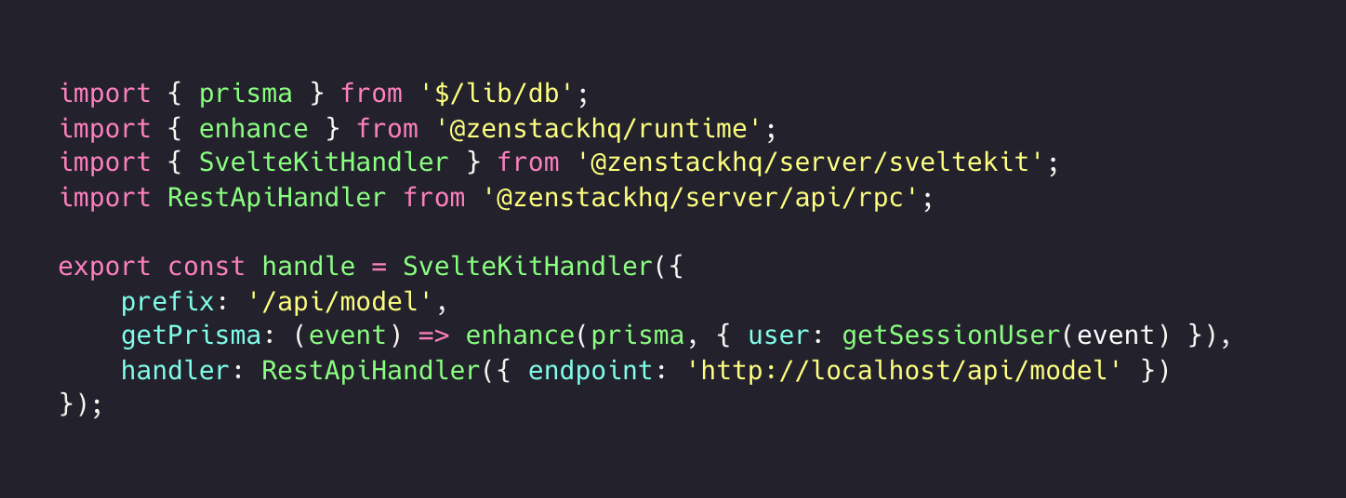
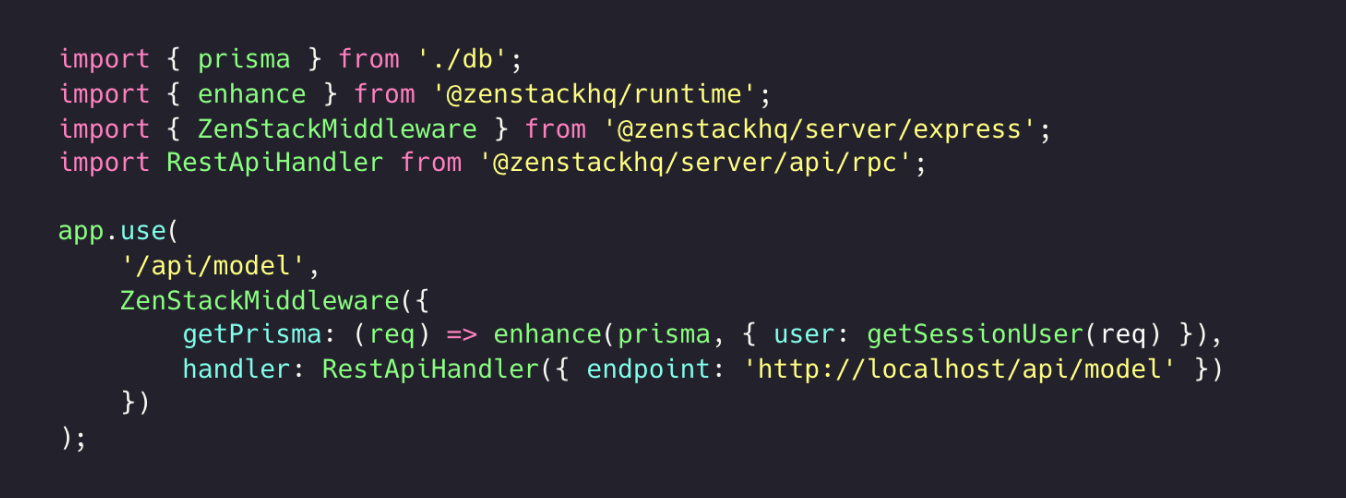
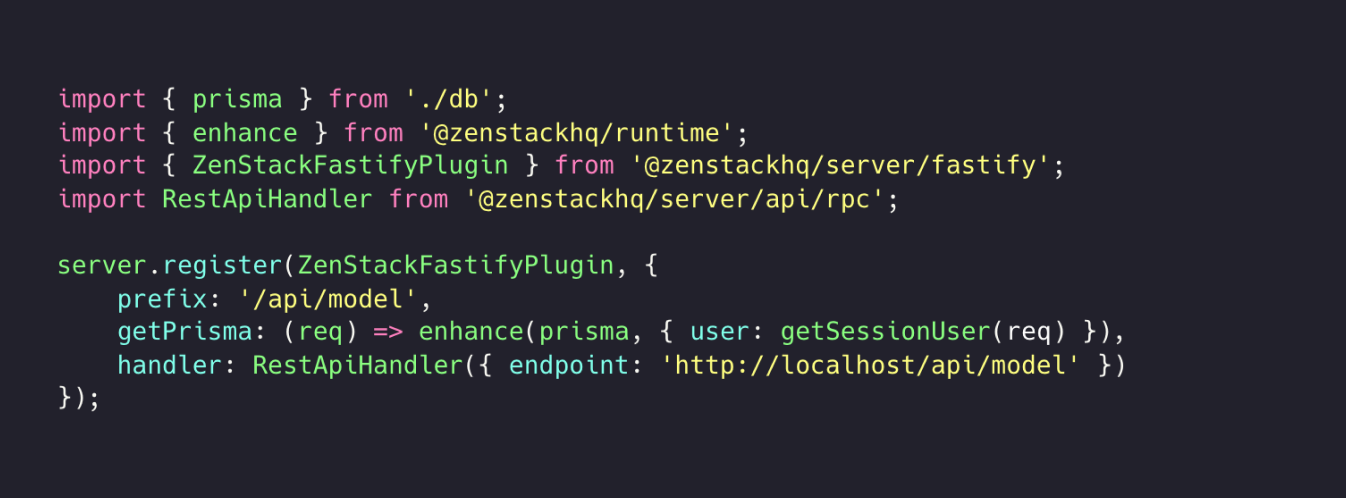
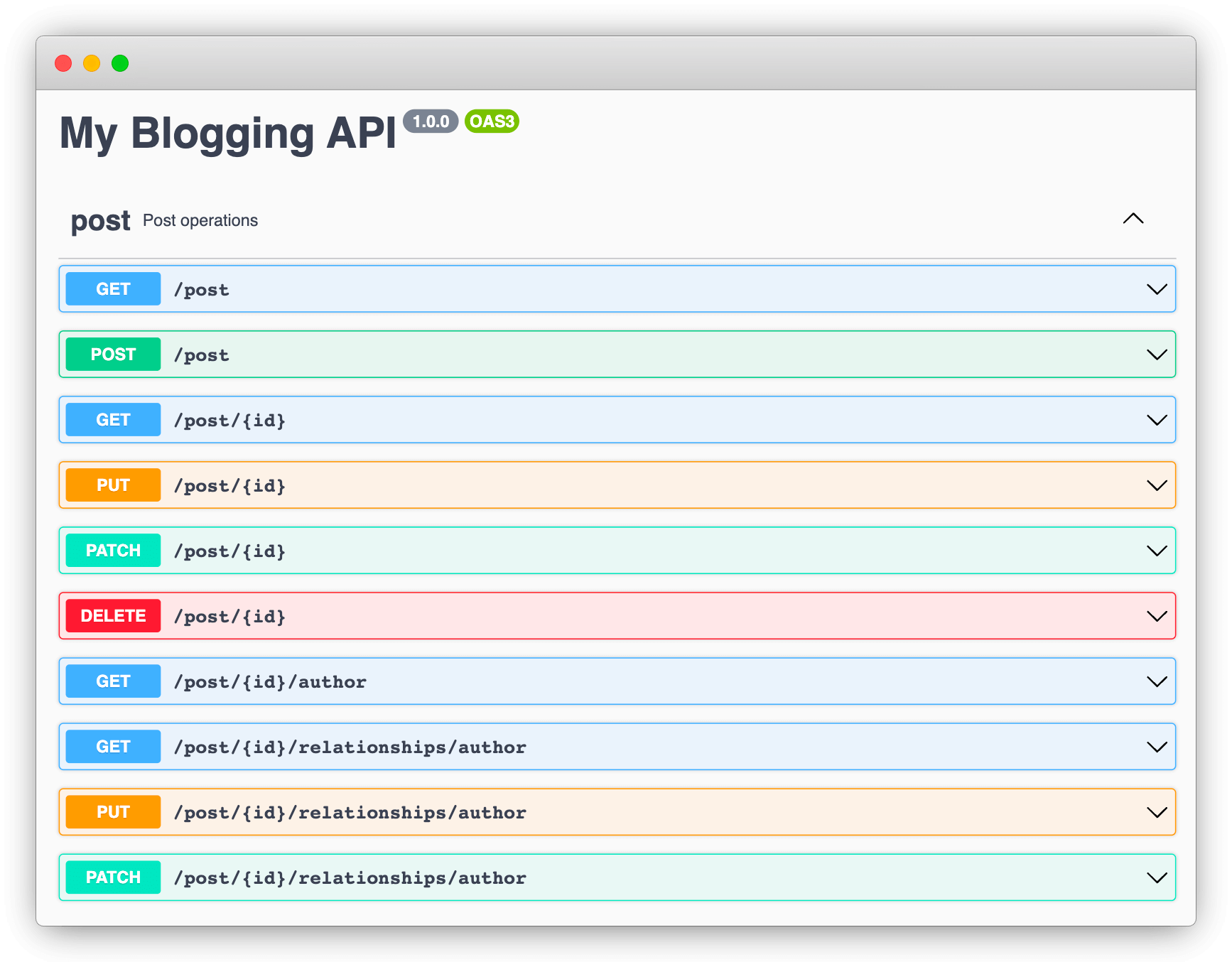
Frontend Query Code Generation
Data query and mutation are one of the toughest topics for frontend development. ZenStack simplifies it by generating fully-typed client-side data access code (aka hooks) targeting the data query library of your choice (SWR, TanStack Query, etc.). The hooks call into the automatically generated APIs, which are secured by the access policies.
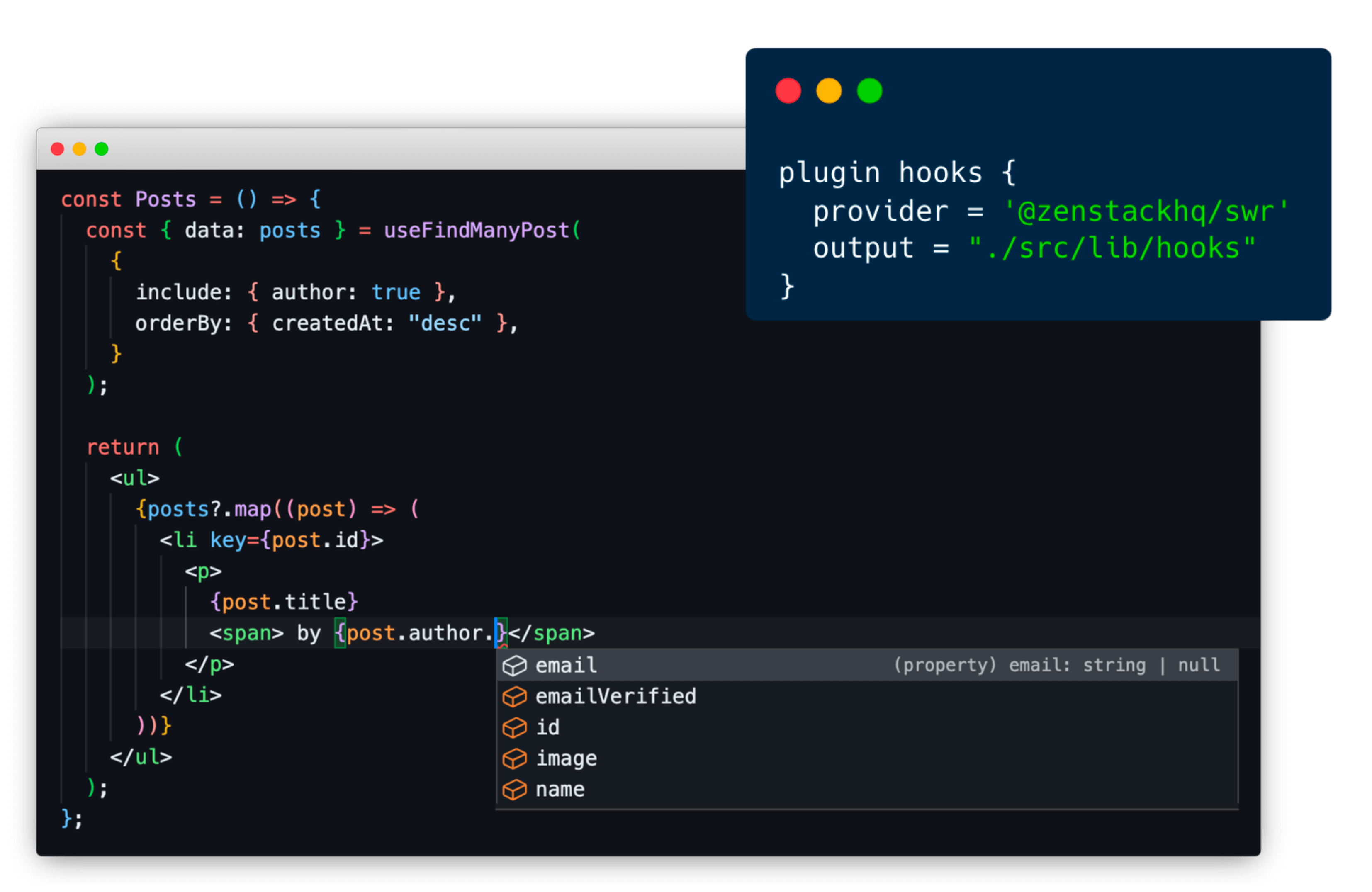
Integrated With The Tools You Love
Server & Full-stack
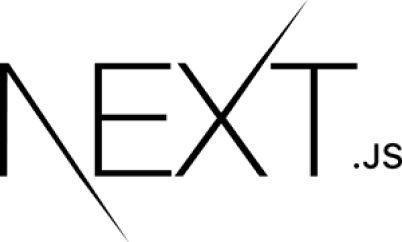
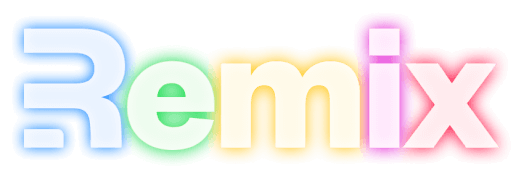
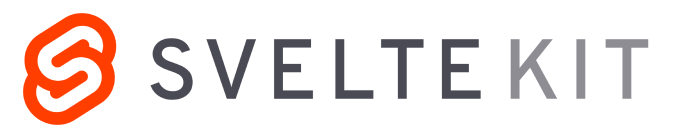
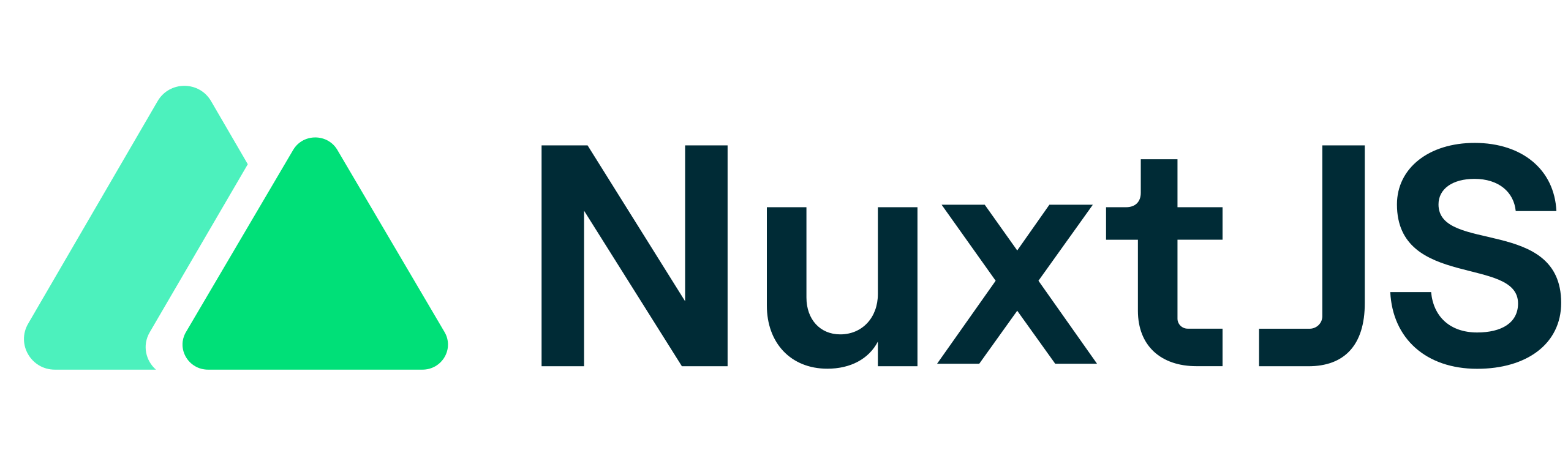
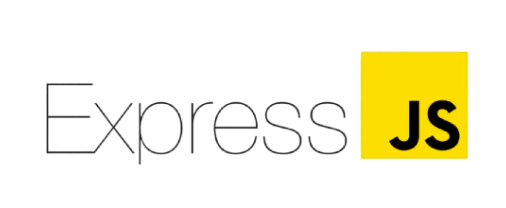
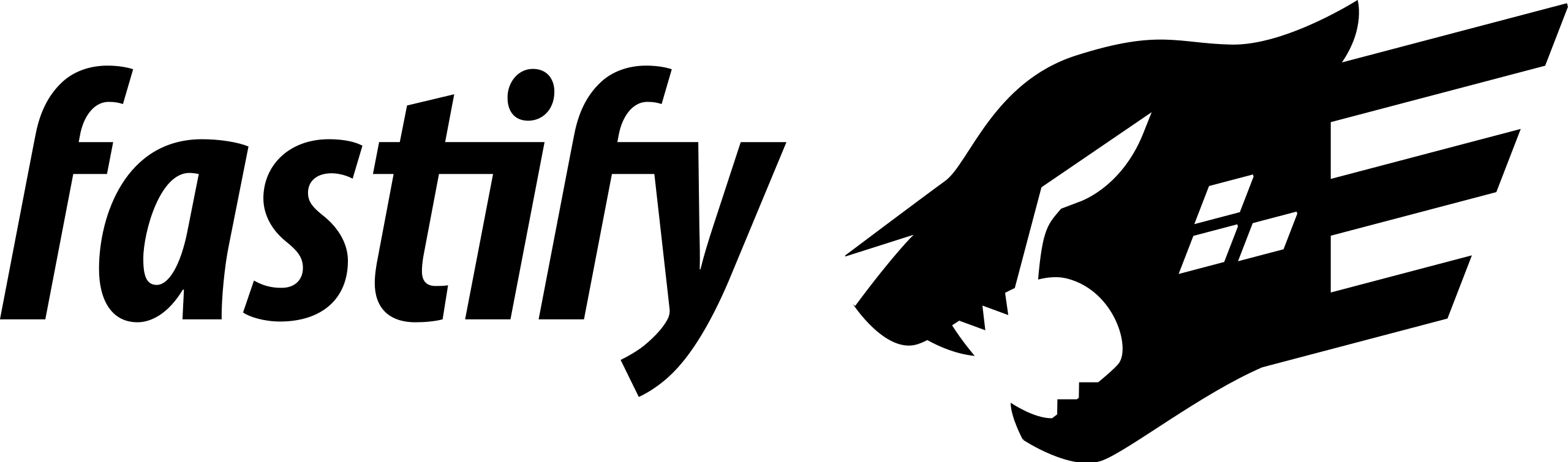
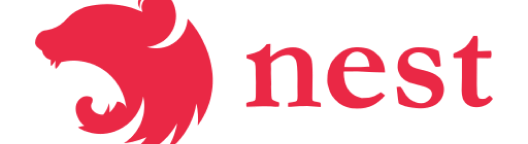
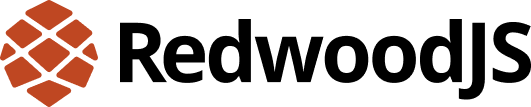
Data Query Client
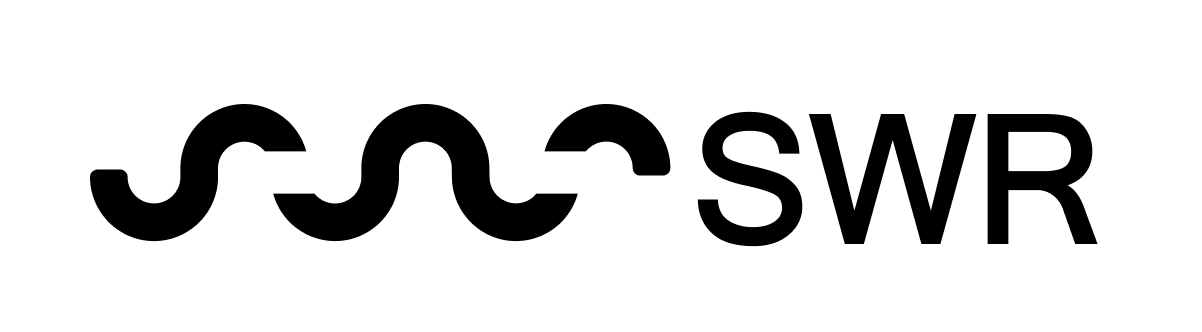

API
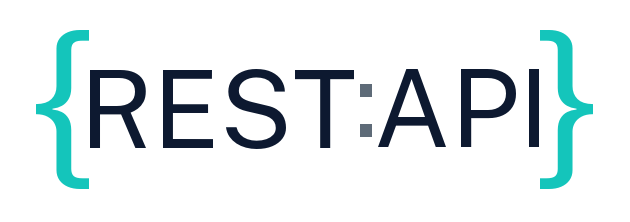
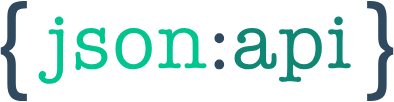
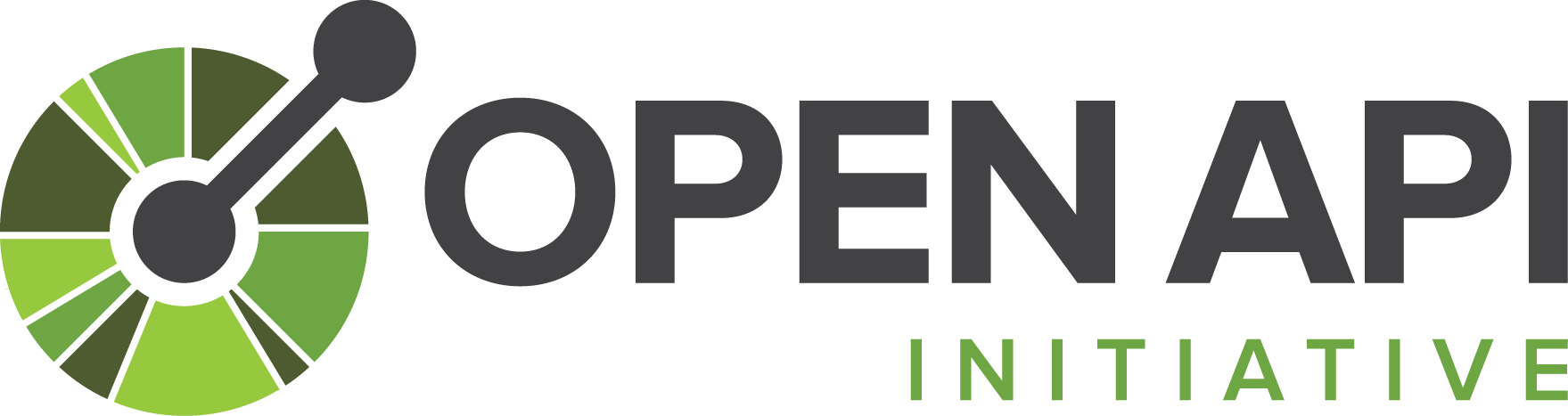
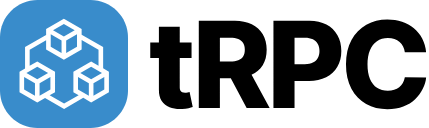
Our Generous Sponsors
Voice of Developers
Thanks for creating such a wonderful product! It was a breeze to adopt, and everyone in the team loves how easy writing the policies are.
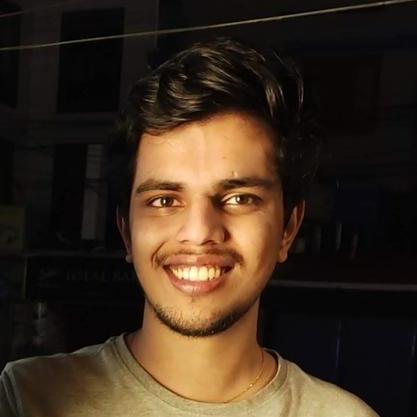
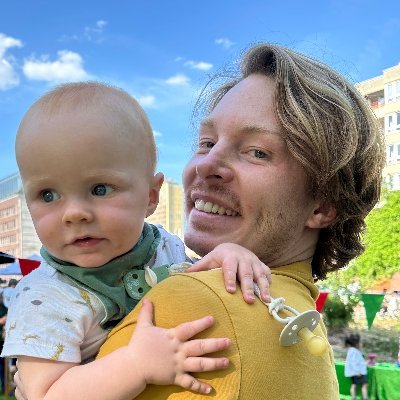
👀 This project by @jiashenggo and @ymcao9 looks really interesting!
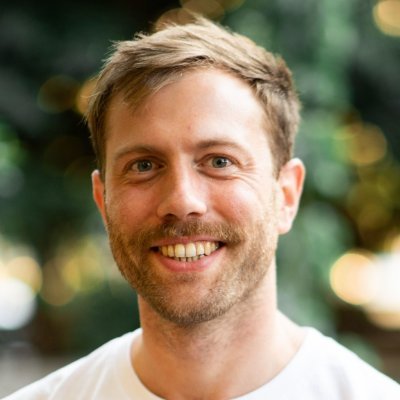
Like REALLY nice
Maybe the future
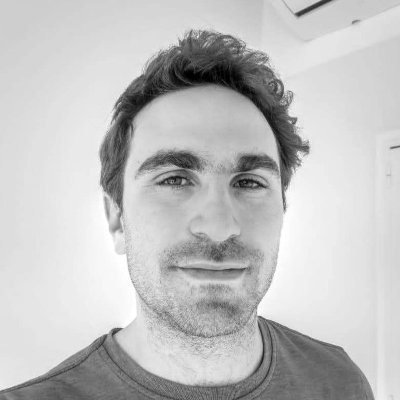
🤯 This is really crazy.
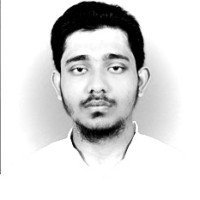
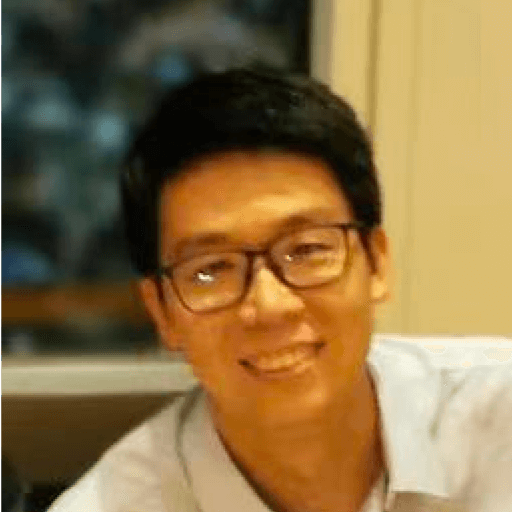